Seleniumを使うことで、ブラウザ操作を自動化できます。例えば、Webフォームにデータを入力したり、ボタンをクリックするなどの自動入力などをすることができます。
今回は様々な場面でPythonのseleniumの使い方を説明します。
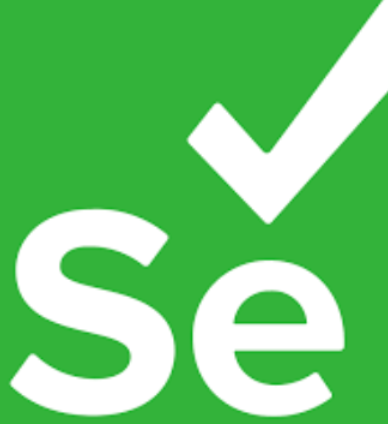
本記事の内容
フォームの要素を取得して文字を入力する
find_element() を使ってフォームの要素(Name)を取得し、send_keys() で入力します。
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get("https://example.com/login")
# フォーム入力
driver.find_element(By.NAME, "username").send_keys("testuser")
driver.find_element(By.NAME, "password").send_keys("password123")
ボタンをクリックする
click()を使ってボタンをクリックします。要素(Name)ではなくXPATHで指定することもできます。
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get("https://example.com")
# ボタンをクリック
driver.find_element(By.XPATH, "//button[text()='ログイン']").click()
driver.find_element(By.NAME, "submit").click()
ページ遷移を待つ+要素を取得する
timeを使ってクリック後のぺージ遷移を待ちます。
さらに、Class名を指定して要素を取得します、文字列を.textで表示します。
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
driver = webdriver.Chrome()
driver.get("https://example.com")
# リンクをクリック
driver.find_element(By.LINK_TEXT, "次のページへ").click()
time.sleep(2) # ページ遷移を待つ
# 遷移後のページで要素を取得
element = driver.find_element(By.CLASS_NAME, "target-element")
print(element.text)
ファイルをダウンロードする(ダウンロードするパスを指定)
URLを指定してファイルをダウンロードします。ファイルダウンロードするパスをあらかじめ指定しておきます。
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_experimental_option("prefs", {
"download.default_directory": "/path/to/download"
})
driver = webdriver.Chrome(options=chrome_options)
driver.get("https://example.com/download")
# ダウンロードボタンをクリック
driver.find_element(By.CLASS_NAME, "download-button").click()
正規表現でURLを抽出する
リンク先がjavascriptで指定されている場合に、「location.href=<URL>」のような文字列が取得できます。
さらにそのURLを抽出するために正規表現を使ってます。
from selenium import webdriver
from selenium.webdriver.common.by import By
import re
driver = webdriver.Chrome()
driver.get("https://example.com/download-page")
# onclick属性を取得
onclick_value = driver.find_element(By.XPATH, "//button[@id='download']").get_attribute("onclick")
# URLを抽出
match = re.search(r"location\.href='(.*?)'", onclick_value)
if match:
download_url = match.group(1)
print(f"ダウンロードURL: {download_url}")
JavaScriptで動的に生成される要素を操作する
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get("https://example.com")
# JavaScriptで動的に表示される要素を待つ
element = driver.find_element(By.ID, "dynamic-content")
print(element.text)
Webページのアラートを処理する
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com")
# アラートを処理
alert = driver.switch_to.alert
print(alert.text)
alert.accept()
エラーをキャッチし、デバッグする
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
driver = webdriver.Chrome()
driver.get("https://example.com")
try:
element = driver.find_element(By.CLASS_NAME, "non-existent")
except NoSuchElementException:
print("要素が見つかりませんでした。")
これらの方法は基本的な操作ですが、さらに応用すれば、様々なフォームを自動入力できます。
今回は以上です。
コメント